Optimizing Database Queries for High-Performance Applications
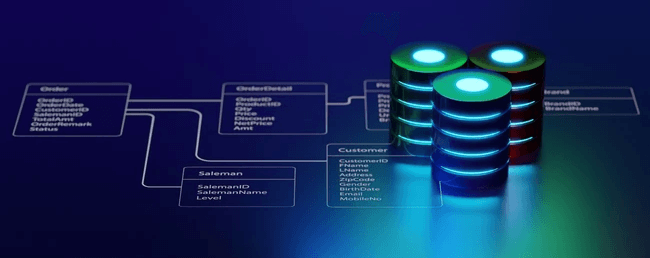
Efficient database query design is crucial for building applications that scale effectively. Whether you're dealing with a high-traffic web application or a data-intensive backend service, poorly optimized queries can become a bottleneck that hampers performance. In this guide, we'll explore several techniques to optimize your database queries, focusing on both SQL and NoSQL databases, and offer practical examples for each.
Why Query Optimization Matters
Inefficient queries can lead to high latency, slow response times, and unnecessary load on your database. These issues are magnified in applications with large user bases or complex data models. Optimizing queries can drastically improve your application's scalability, reduce server load, and enhance the user experience.
General Tips for Query Optimization
1. Use Indexes Effectively
Indexes are one of the most powerful tools for speeding up database queries. They allow the database to quickly locate rows that match certain criteria, without having to scan the entire table. However, using indexes improperly can actually slow down your application.
- Use indexes on columns that are frequently queried: For instance, fields involved in
WHERE
,JOIN
,ORDER BY
, orGROUP BY
clauses are good candidates for indexing. - Avoid over-indexing: Each index comes with an overhead in terms of storage and performance during inserts or updates. Too many indexes can slow down write operations.
Example:
CREATE INDEX idx_user_email ON users(email);
2. Limit the Data You Retrieve
Instead of retrieving all columns with a SELECT *
, specify only the fields you actually need. This reduces the amount of data transferred from the database to your application.
Example:
SELECT id, name, email FROM users WHERE status = 'active';
This query only retrieves the id
, name
, and email
columns, reducing the load on the database and network.
3. Optimize Joins
Joins are essential in many queries, but they can be performance-intensive, especially with large datasets. To optimize joins:
- Use the appropriate type of join: Use
INNER JOIN
when you only need matching rows from both tables. Avoid unnecessaryLEFT JOIN
if you don't need all rows from the left table. - Ensure indexed columns are being joined: Joining on indexed columns can significantly improve performance.
Example:
SELECT u.name, p.product_name FROM users u INNER JOIN purchases p ON u.id = p.user_id WHERE p.status = 'completed';
In this example, both the users.id
and purchases.user_id
columns should be indexed for optimal performance.
4. Avoid N+1 Query Problems
The N+1 query problem occurs when you retrieve data in a loop, causing multiple database queries. This is inefficient, especially when working with large datasets. Instead, use techniques like batch queries or joins to minimize the number of database calls.
Example (N+1 Query Problem):
// Fetching posts and comments one by one for (let post of posts) { let comments = db.query('SELECT * FROM comments WHERE post_id = ?', [post.id]); }
Instead, optimize by fetching all necessary data in one query:
// Optimized query to fetch posts and comments in one go let result = db.query('SELECT p.title, c.comment FROM posts p LEFT JOIN comments c ON p.id = c.post_id');
5. Use Pagination for Large Result Sets
When querying large datasets, avoid retrieving the entire result set in one go. Instead, implement pagination to fetch data in smaller, more manageable chunks.
Example:
SELECT * FROM users ORDER BY created_at LIMIT 10 OFFSET 0; -- Fetch first 10 users
This approach helps reduce memory usage and improves query response time.
Advanced Optimization Techniques
1. Query Caching
Many databases support query caching, which stores the results of frequently executed queries in memory. When the same query is executed again, the database can retrieve the cached result instead of running the query again, significantly speeding up response times.
- MySQL: MySQL has built-in query caching (though it’s being deprecated in newer versions).
- PostgreSQL: PostgreSQL does not have query caching, but you can use external caching solutions like Redis or Memcached to cache query results.
2. Optimize Subqueries
Subqueries can be slow, especially when they’re executed repeatedly. Consider replacing subqueries with joins, CTEs (Common Table Expressions), or window functions when possible.
Example (Subquery):
SELECT name FROM users WHERE id IN (SELECT user_id FROM orders WHERE status = 'completed');
Optimized with a JOIN
:
SELECT u.name FROM users u INNER JOIN orders o ON u.id = o.user_id WHERE o.status = 'completed';
3. Batch Updates and Inserts
If your application requires frequent inserts or updates, consider batching multiple operations into a single query to reduce database load.
Example:
-- Inserting multiple rows in one query INSERT INTO orders (user_id, product_id, quantity) VALUES (1, 101, 2), (2, 102, 3), (3, 103, 1);
Batching reduces the number of database round trips, which can result in significant performance improvements.
4. Using Database Partitioning
For very large datasets, partitioning can help split a large table into smaller, more manageable pieces. Each partition can be stored on different physical storage, allowing faster query execution for specific subsets of data.
Example (Partitioning in MySQL):
CREATE TABLE orders ( id INT, order_date DATE, ... ) PARTITION BY RANGE (YEAR(order_date)) ( PARTITION p2019 VALUES LESS THAN (2020), PARTITION p2020 VALUES LESS THAN (2021), PARTITION p2021 VALUES LESS THAN (2022) );
Partitioning helps ensure that queries on specific date ranges are more efficient.
NoSQL Query Optimization
For NoSQL databases like MongoDB, optimization techniques can differ from traditional relational databases:
- Indexing: Like SQL, NoSQL databases allow indexes on frequently queried fields. In MongoDB, use
db.collection.createIndex()
for efficient searches. - Denormalization: In NoSQL databases, consider denormalizing data by storing redundant copies of data, which can reduce the need for joins.
- Sharding: Sharding distributes data across multiple servers, enabling horizontal scaling.
Example of creating an index in MongoDB:
db.users.createIndex({ email: 1 });
Conclusion
Optimizing database queries is crucial for building high-performance, scalable applications. Whether you're using SQL or NoSQL databases, the techniques outlined in this guide—such as indexing, efficient joins, pagination, and query caching—can help you significantly improve query performance.
By following these practices, you'll be able to handle larger datasets, reduce latency, and scale your application more effectively. Always analyze your query execution plans, identify bottlenecks, and continuously optimize based on your application’s needs.
Happy coding!
This article provides a comprehensive overview of query optimization techniques for databases, offering practical tips and examples that are directly applicable to your backend development projects.
Tags
Related Posts
About the Author
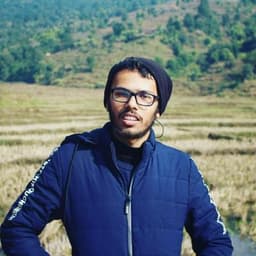
Sagar Subedi
A passionate writer and technologist exploring the intersections of code and creativity.